Crank Up Your AI Skills in Minutes with This Lightning-Fast Python Crash Course!
10 min read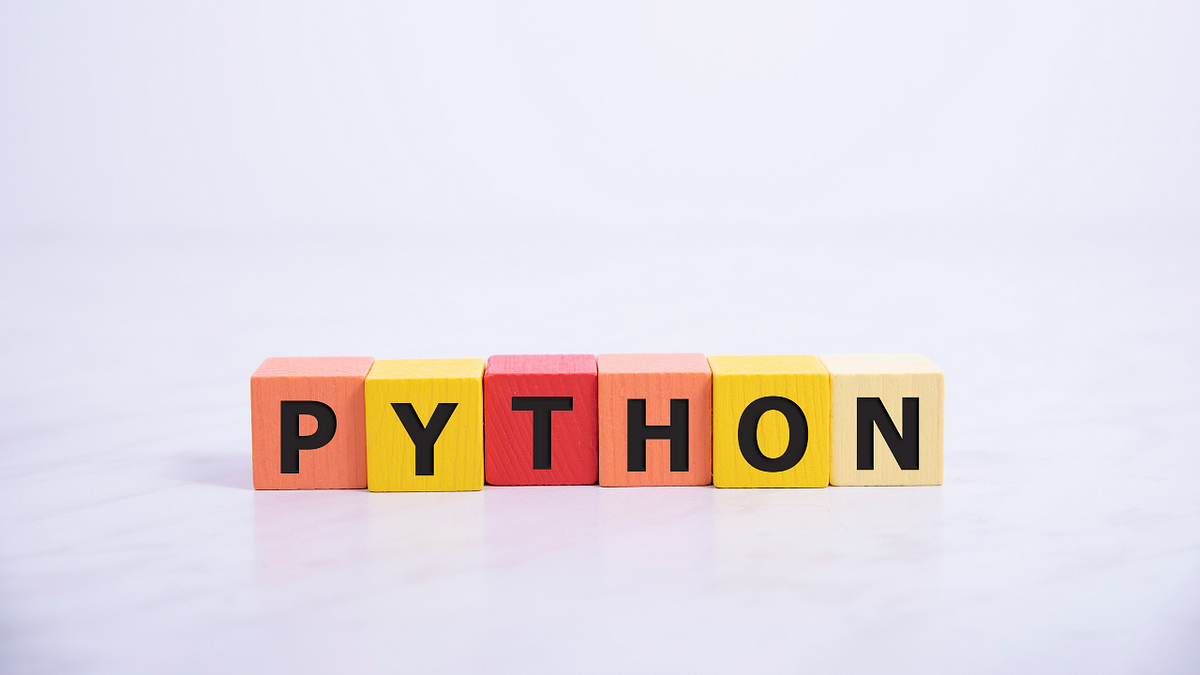
Checking for Pre-Installed Python
Many computers come with Python pre-installed. To verify this, navigate to your Terminal (on Mac or Linux) or Command Prompt (on Windows). Simply type “python” into the command line interface and press Enter. If Python is installed on your machine, you should see its version number displayed in response.
Using Python in Terminal. Image by author.
Setting Up Your Python Environment
If you don’t see a screen like this, you can download Python manually from the official website (Windows / Mac). Alternatively, one can install Anaconda, a popular Python package system for AI and data science. If you run into installation issues, ask your favorite AI assistant for help!
Getting Started with Python Programming
With Python running, you’re now ready to start writing some code. It’s recommended that you run the examples on your computer as we go along. You can also download all the example code from the GitHub repo.
Strings & Numbers
Data Types: The Foundation of Efficient Computing
In the world of computing, data types play a crucial role in determining how data is processed and utilized by machines. A data type, or simply “type”, is a way to categorize data so that it can be handled effectively and efficiently within a computer.
By classifying data into specific categories, computers are able to perform tasks such as storing, retrieving, and manipulating the data in an organized and meaningful manner. This classification system allows for the creation of software applications that can handle various types of data with ease, making computing more efficient and productive.
Understanding Types in Programming
In programming, types are defined by a possible set of values and operations. A type is essentially a classification of data that determines how it should be treated by the program.
For instance, strings are a type of sequence of characters, known as arbitrary character sequences or text. This type can be manipulated using specific operations, such as concatenation, substring extraction, and searching.
To illustrate this concept further, you can try out some string-related commands in your command line Python instance.
"this is a string"
>> 'this is a string'
'so is this:-1*!@&04"(*&^}":>?'
>> 'so is this:-1*!@&04"(*&^}":>?'
"""and
this is
too!!11!"""
>> 'and\n this is\n too!!11!'
"we can even " + "add strings together"
>> 'we can even add strings together'
Although strings can be added together (i.e. concatenated), they can’t be added to numerical data types like int (i.e. integers) or float (i.e. numbers with decimals). If we try that in Python, we will get an error message because operations are only defined for compatible types.
# we can't add strings to other data types (BTW this is how you write comments in Python)
"I am " + 29
>> TypeError: can only concatenate str (not "int") to str
# so we have to write 29 as a string
"I am " + "29"
>> 'I am 29'
Lists & Dictionaries
Beyond the basic types of strings, ints, and floats, Python has types for structuring larger collections of data.
One such type is a list, an ordered collection of values. We can have lists of strings, numbers, strings + numbers, or even lists of lists.
# a list of strings
["a", "b", "c"]
# a list of ints
[1, 2, 3]
# list with a string, int, and float
["a", 2, 3.14]
# a list of lists
[["a", "b"], [1, 2], [1.0, 2.0]]
Another core data type is a dictionary, which consists of key-value pair sequences where keys are strings and values can be any data type. This is a great way to represent data with multiple attributes.
# a dictionary
{"Name":"Shaw"}
# a dictionary with multiple key-value pairs
{"Name":"Shaw", "Age":29, "Interests":["AI", "Music", "Bread"]}
# a list of dictionaries
[{"Name":"Shaw", "Age":29, "Interests":["AI", "Music", "Bread"]},
{"Name":"Ify", "Age":27, "Interests":["Marketing", "YouTube", "Shopping"]}]
# a nested dictionary
{"User":{"Name":"Shaw", "Age":29, "Interests":["AI", "Music", "Bread"]},
"Last_login":"2024-09-06",
"Membership_Tier":"Free"}
So far, we’ve seen some basic Python data types and operations. However, we are still missing an essential feature: variables.
Variables: An Abstract Representation of Data
Variables provide an abstract representation of an underlying data type instance, allowing us to assign a value to a named location in our program. For instance, we can create a variable called user_name
, which represents a string containing the text “Shaw”. This enables us to write flexible programs that are not limited to specific values.
By using variables, we can decouple our code from specific data and make it more reusable and maintainable. Variables also allow us to manipulate and transform data in a programmatic way, making them an essential concept in programming languages.
# creating a variable and printing it
user_name = "Shaw"
print(user_name)
#>> Shaw
We can do the same thing with other data types e.g. ints and lists.
# defining more variables and printing them as a formatted string.
user_age = 29
user_interests = ["AI", "Music", "Bread"]
print(f"{user_name} is {user_age} years old. His interests include {user_interests}.")
#>> Shaw is 29 years old. His interests include ['AI', 'Music', 'Bread'].
Writing and Executing Sophisticated Programs from the Command Line
As our code snippets continue to grow in complexity, it’s essential to learn how to write and execute more advanced scripts from the command line. This will allow us to automate tasks and streamline workflows with ease.
To get started, let’s focus on creating our first script. We’ll explore the process of writing and executing sophisticated programs from the command line, giving you a solid foundation for more complex programming endeavors.
Getting Started with Python
Create a new folder on your computer, which I will refer to as python-quickstart
. Open this folder using your preferred IDE (e.g., Integrated Development Environment) and create a new Python file, for example, my-script.py
.
In this new file, we can write the classic “Hello, world” program.
# ceremonial first program
print("Hello, world!")
If you don’t have an IDE (not recommended), you can use a basic text editor (e.g. Apple’s Text Edit, Window’s Notepad). In those cases, you can open the text editor and save a new text file using the .py extension instead of .txt. Note: If you use TextEditor on Mac, you may need to put the application in plain text mode via Format > Make Plain Text.
We can then run this script using the Terminal (Mac/Linux) or Command Prompt (Windows) by navigating to the folder with our new Python file and running the following command.
python my-script.py
Congrats! You ran your first Python script. Feel free to expand this program by copy-pasting the upcoming code examples and rerunning the script to see their outputs.
4) Loops and Conditions
Two fundamental functionalities of Python (or any other programming language) are loops and conditions.
Loops allow us to run a particular chunk of code multiple times. The most popular is the for loop, which runs the same code while iterating over a variable.
# a simple for loop iterating over a sequence of numbers
for i in range(5):
print(i) # print ith element
# for loop iterating over a list
user_interests = ["AI", "Music", "Bread"]
for interest in user_interests:
print(interest) # print each item in list
# for loop iterating over items in a dictionary
user_dict = {"Name":"Shaw", "Age":29, "Interests":["AI", "Music", "Bread"]}
for key in user_dict.keys():
print(key, "=", user_dict[key]) # print each key and corresponding value
The other core function is conditions, such as if-else statements, which enable us to program logic. For example, we may want to check if the user is an adult or evaluate their wisdom.
# check if user is 18 or older
if user_dict["Age"] >= 18:
print("User is an adult")
# check if user is 1000 or older, if not print they have much to learn
if user_dict["Age"] >= 1000:
print("User is wise")
else:
print("User has much to learn")
It’s common to use conditionals within for loops to apply different operations based on specific conditions, such as counting the number of users interested in bread.
# count the number of users interested in bread
user_list = [{"Name":"Shaw", "Age":29, "Interests":["AI", "Music", "Bread"]},
{"Name":"Ify", "Age":27, "Interests":["Marketing", "YouTube", "Shopping"]}]
count = 0 # intialize count
for user in user_list:
if "Bread" in user["Interests"]:
count = count + 1 # update count
print(count, "user(s) interested in Bread")
Functions are operations we can perform on specific data types
Useful Functions in Python
We’ve already explored the print()
function, which can be used with any data type. But there are several other useful functions in Python that are worth learning about.
These functions make it easier to work with your code and provide more flexibility when needed. In this article, we’ll take a closer look at some of these essential functions.
# print(), a function we've used several times already
for key in user_dict.keys():
print(key, ":", user_dict[key])
# type(), getting the data type of a variable
for key in user_dict.keys():
print(key, ":", type(user_dict[key]))
# len(), getting the length of a variable
for key in user_dict.keys():
print(key, ":", len(user_dict[key]))
# TypeError: object of type 'int' has no len()
We see that, unlike print()
and type()
, len()
is not defined for all data types, so it throws an error when applied to an int. There are several other type-specific functions like this.
# string methods
# --------------
# make string all lowercase
print(user_dict["Name"].lower())
# make string all uppercase
print(user_dict["Name"].upper())
# split string into list based on a specific character sequence
print(user_dict["Name"].split("ha"))
# replace a character sequence with another
print(user_dict["Name"].replace("w", "whin"))
# list methods
# ------------
# add an element to the end of a list
user_dict["Interests"].append("Entrepreneurship")
print(user_dict["Interests"])
# remove a specific element from a list
user_dict["Interests"].pop(0)
print(user_dict["Interests"])
# insert an element into a specific place in a list
user_dict["Interests"].insert(1, "AI")
print(user_dict["Interests"])
# dict methods
# ------------
# accessing dict keys
print(user_dict.keys())
# accessing dict values
print(user_dict.values())
# accessing dict items
print(user_dict.items())
# removing a key
user_dict.pop("Name")
print(user_dict.items())
# adding a key
user_dict["Name"] = "Shaw"
print(user_dict.items())
While the core Python functions are helpful, the real power comes from creating user-defined functions to perform custom operations. Additionally, custom functions allow us to write much cleaner code. For example, here are some of the previous code snippets repackaged as user-defined functions.
# define a custom function
def user_description(user_dict):
# Function to return a sentence (string) describing input user
return f'{user_dict["Name"]} is {user_dict["Age"]} years old and is interested in {user_dict["Interests"][0]}.'
# print user description
description = user_description(user_dict)
print(description)
# print description for a new user!
new_user_dict = {"Name":"Ify", "Age":27, "Interests":["Marketing", "YouTube", "Shopping"]}
print(user_description(new_user_dict))
# define another custom function
def interested_user_count(user_list, topic):
# Function to count number of users interested in an arbitrary topic
count = 0
for user in user_list:
if topic in user["Interests"]:
count = count + 1
return count
# define user list and topic
user_list = [user_dict, new_user_dict]
topic = "Shopping"
# compute interested user count and print it
count = interested_user_count(user_list, topic)
print(f"{count} user(s) interested in {topic}")
Python’s strength lies in its ability to quickly implement complex tasks due to its vibrant developer community and robust ecosystem of software packages. In many cases, you won’t need to spend time building a program from scratch because the solution likely already exists as an open-source library. Python’s vast repository of libraries makes it an ideal choice for rapid development, allowing developers to focus on the logic of their application rather than reinventing the wheel.
Installing Packages with Python’s Native Package Manager, pip
We can install such packages using Python’s native package manager, pip. To install new packages, we run pip commands from the command line.
To install an essential data science library, such as numpy, we follow these steps:
Install numpy using pip
- Open a terminal or command prompt.
- Run the following command:
pip install numpy
- Wait for the installation to complete.
After completing the installation, you can verify that numpy is installed by running import numpy
in your Python environment.
pip install numpy
After we’ve installed numpy, we can import it into a new Python script and use some of its data types and functions.
import numpy as np
# create a "vector"
v = np.array([1, 3, 6])
print(v)
# multiply a "vector"
print(2*v)
# create a matrix
X = np.array([v, 2*v, v/2])
print(X)
# matrix multiplication
print(X*v)
The previous pip command added numpy to our base Python environment. Alternatively, it’s a best practice to create so-called virtual environments. These are collections of Python libraries that can be readily interchanged for different projects.
Here’s how to create a new virtual environment called my-env.
python -m venv my-env
Then, we can activate it.
# mac/linux
source my-env/bin/activate
# windows
.\my-env\Scripts\activate.bat
Finally, we can install new libraries, such as numpy, using pip.
pip install pip
Note: If you’re using Anaconda, check out this handy cheatsheet for creating a new conda environment.
Several other libraries are commonly used in AI and data science. Here is a non-comprehensive overview of some helpful ones for building AI projects.
Example Code: Extracting summary and keywords from research papers
Now that we have been exposed to the basics of Python, let’s see how we can use it to implement a simple AI project. Here, I will use the OpenAI API to create a research paper summarizer and keyword extractor.
Like all the other snippets in this guide, the example code is available at the GitHub repository.