4 tricks to writing modern Python
7 min read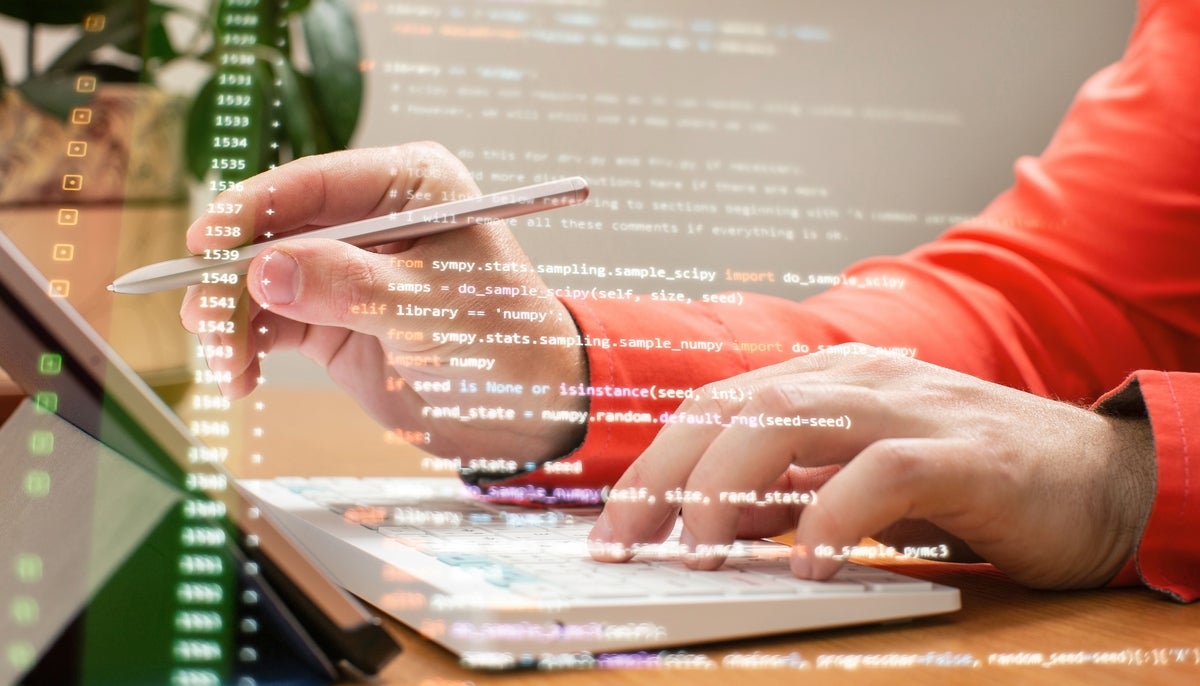
Although Python had its 30-year anniversary in 2021, the explosion of fostering, development, and forward-thinking advancement related to the language is still reasonably new. Many attributes of Python have actually continued to be unmodified considering that its inception, but with every passing year, and every new edition of Python, there are new methods of doing things and brand-new libraries that benefit from those breakthroughs.
So, Python has its old ways and its new ways. Naturally, it makes good sense to find out exactly how to function with Python using its most modern and hassle-free features. Below, we’ll diminish the key principles you require to recognize to compose contemporary Python in 2024– software application that utilizes Python’s most current and best idioms, principles, and abilities.
Kind hinting in Python
Python’s lately introduced kind hinting phrase structure permits linters and third-party code quality tools to evaluate your code prior to runtime, and to detect possible errors prior to they buzz out. The more you create Python code to show to others, the more probable you (and every person else!) will take advantage of utilizing type tips.
Each succeeding modification of Python turns out much more innovative and effective type annotations. If you get right into the routine of finding out exactly how to make use of type comments in the brief run, you will certainly be much better outfitted to take advantage of each brand-new type hinting development as it’s introduced.
It is necessary to keep in mind that type tips are optional, not mandatory. Not every job needs them. Usage type tips to make your bigger tasks comprehensible, however feel complimentary to omit them from a 50-line offhand manuscript. And, while type tips are not imposed at runtime, you can use Pydantic to make that possible. Lots of commonly made use of Python projects use Pydantic thoroughly– FastAPI is one example.
Python digital atmospheres and package management
For simple jobs and undemanding growth tasks, you can typically just utilize Python’s integrated venv tool to keep jobs and their requirements separate. However recent advancements in Python’s tooling provide you a lot more choices:
Pyenv: If you need to keep several variations of Python mounted to satisfy different job needs, Pyenv allows you switch over in between them either around the world or on a per-project basis. It’s valuable if you locate on your own doing a whole lot of work with various Python versions right at the command line, outside of the context of a per-project online setting. Note that there is no main Windows support, yet an unofficial Windows port does exist.
: If you require to keep numerous variations of Python mounted to satisfy various job needs, Pyenv lets you change between them either worldwide or on a per-project basis. It serves if you locate yourself doing a great deal of collaborate with various Python versions right at the command line, beyond the context of a per-project virtual atmosphere. Note that there is no official Windows assistance, but an informal Windows port does exist. Pipenv: Billed as “Python dev operations for humans,” Pipenv is implied to take care of a virtual atmosphere plus all the dependences for your job. It likewise makes certain dependencies are deterministic, suggesting you get the particular variations you want, and that they operate in the combination you request. Pipenv does not, nevertheless, talk with packaging in any kind, so it’s not perfect for jobs you ultimately wish to upload to PyPI or share with others.
: Billed as “Python dev process for people,” Pipenv is suggested to manage a virtual setting plus all the dependences for your project. It likewise guarantees dependencies are deterministic, implying you obtain the certain versions you want, and that they operate in the combination you ask for. Pipenv does not, nonetheless, speak with product packaging in any type of kind, so it’s not excellent for jobs you eventually wish to post to PyPI or show others. Poetry: Expanding on Pipenv’s toolset, Poetry not just manages tasks and needs, however also makes it simple to release the task to PyPI. It likewise manages virtual environments for you separate from your job directory sites.
: Increasing on Pipenv’s toolset, Poetry not just manages tasks and demands, yet additionally makes it simple to deploy the project to PyPI. It additionally takes care of online atmospheres for you divide from your task directories. PDM: PDM (short for Python Advancement Master) is a current cutting-edge project in this blood vessel. Like Verse and Pipenv, PDM gives you with a single user interface for establishing a job, handling its reliances, and building distribution artefacts from it. PDM additionally utilizes the PEP 582 requirement for storing bundles in your area to a project, so there is no need to create per-project online settings. However this tool is relatively brand-new, so make certain it functions provisionally before embracing it in production.
: PDM (short for Python Advancement Master) is a current cutting-edge task in this capillary. Like Poetry and Pipenv, PDM offers you with a single user interface for setting up a job, handling its dependencies, and structure distribution artefacts from it. PDM additionally utilizes the PEP 582 requirement for keeping bundles locally to a job, so there is no demand to create per-project online settings. Yet this device is fairly new, so make certain it functions provisionally before embracing it in production. Hatch: The hatch task not only manages task setup and monitoring, yet also provides a construct system, devices for packaging jobs for redistribution on PyPI, examination handling, and lots of other helpful features.
: The hatch job not just takes care of project configuration and administration, but additionally provides a develop system, tools for product packaging projects for redistribution on PyPI, test handling, and several other useful features. uv: The speculative uv task is created by the exact same people that make the ruff Python linting tool. It aims to replace pip, venv, and a number of various other command-line Python tools at once. It’s composed in Corrosion for rate (like ruff ), and a lot of its commands appear like those of pip and other devices it replaces, making it fairly very easy to find out.
When producing new projects that are implied to be worked on in a team environment or distributed to others (e.g., using PyPI), make certain to use the modern pyproject.toml format for your needs and job configuration, in addition to the job layout used with it. You can still utilize the older requirements.txt data side-by-side with pyproject.toml, however the last covers a broader array of use situations and makes your jobs forward-compatible.
New Python syntax
Python’s evolution has meant many new enhancements to the language itself. The last couple of versions of Python have actually added useful syntactical constructions that enable extra effective and succinct progamming. While they aren’t compulsory, newer third-pary modules might use them, so they deserve being familiar with at the very least delicately.
3 recent phrase structure additions are particularly remarkable.
Pattern matching
The largest recent enhancement, architectural pattern matching, which showed up in Python 3.10, is more than just” switch/case for Python” as it has often been described. Architectural pattern matching allows you make control-flow decisions based on the contents or structure of things. Basically, it’s a means to match based upon types or the forms of kinds (a checklist with an int and a string, for example) as opposed to values.
The ‘walrus operator’
So called for its appearance (:= ), the walrus operator, included Python 3.8, introduces task expressions, a way to appoint a value to a variable and afterwards apply a test to the variable in a solitary action. It makes for less verbose code in many typical situations, such as inspecting a feature’s return value while also preserving the outcomes.
Positional-only criteria
A minor but helpful recent enhancement to Python’s syntax, positional-only parameters, allows you show which operate criteria should be defined as positional ones, never ever as keyword disagreements. This feature is generally meant to boost the quality and ease the future advancement of a codebase, objectives that a lot of Python’s various other brand-new features likewise focus on.
Python testing
Writing tests for a codebase resembles flossing day-to-day: Every person agrees it’s an excellent thing, few of us really do it, and even fewer do it correctly. Modern Python codebases be worthy of to have test suites, and the current tooling for testing makes developing examination suites less complicated than ever.
Python has its very own built-in testing structure, unittest. It isn’t negative as a default, but its design and actions are dated. The Pytest structure has actually risen to importance as a common replacement. It’s more flexible (you can state examinations in any kind of component of your code, not just a part) and requires writing much less boilerplate. Plus, Pytest has lots of attachments to broaden its capability (e.g., for testing asynchronous code).
Another important adjunct to screening is code insurance coverage, identifying exactly how much of one’s codebase the tests really cover. The module Coverage has you covered for this (as the name suggests) and Pytest also includes a plug-in to collaborate with it.